Different types of Functions in C Language
Library functions :
These all are set of pre-implemented f’ns which is available along with compiler
- The implementation part of the predefined f’n is available in library files. I.e “.lib” or “.obj” files
- .lib or .obj files contains pre-compiled code.
- When we are working with pre-defined f’ns to avoid the compilation errors, we require to go for headerfiles
- Always headerfiles doesn’t contains any implementation part of predefined f’ns, it contains only prototype.
Limitations :
- All predefined f’ns contains limited task only. i.e for what purpose is implemented, for same purpose it will use.
- As a programmer, we don’t having any controls on pre-defined f’ns, because coding part is not available.
- As a programmer, we can’t alter or modify the behavior of any predefined f’n
- In implemention, whenever a pre-defined f’n is not supporting user requirement , then go for user defined f’n
User defined function : -
Accoring to the project requirement or according to the client requirement , if we implementing any new f’n, it is called user defined f’n As a programmer, we are having full controls on any user defined f’n, because coding part is available in user readable format.
- As a programmer, it is possible to change or alter the behavior of any user defined f’n
- User defined f’ns are classified into “4” types i.e,
- Functions with no arguments are no return value
- Functions with arguments and no return type
- Function with no arguments and one return value.
Note: All pre-defined f’ns are user defined f’ns only because somewhere else another programmer is implemented these f’ns and we are using in the form of object code. So, it became pre-defined f’ns for us.
ABOUT mian() :
- main is an identifier in the program, which indicates startup point of an application.
- Main is a user defined f’n with predefined signature for linker.
- Always main f’n will indicates from which location program need to be starts.
- Without using main f’n , we can implement the program but execution is not possibe. In this case, compilation became success but linking became error.
- It is possible to change the name of the main() f’n, but if we are changing the main, then mandatary to implement with another f’n with the name called main.
- Generally main() f’n doesn’t returns any value that’s why return type of the main f’n is valid.
- In implementation, when we require to provide exit status of an application back to the “OS” then specify the return type as an int.
- void main() doesn’t provides any exit status back to the operating system
- Int main() will provide exit status back to the “os” e success or failure
- In implementation when we need to return the exit status as a success then specify the return value as “0” i.e “return 0”
- Whenever we need to inform the exit status as failure, then return value is “1” i.e “return 1”
- whenever main f’n return type is an int, it is possible to return the value from range of -32768 to +32768 but except 0 and 1 , rest of all exit codes ae meaningless.
- Whenever the user is terminating the program explicitly then exit code is -1
void main()
{ printf(“\n welcome abc”); }
void main() //&main
{ printf(“\n welcome main1”); abc(); // calling &abc(); abc(); // calling &abc(); printf(“\n welcome main2”); } welcome abc welcome abc welcome main2
A ‘c’ program is a collection of predefined and user defiend f’ns
- Always compilation process will takes place from top to bottom, execution process will starts from main and ends with main only.
- In order to compile the program, if any f’ns are occur, then it will generates a unique identification value called f’n address
- At the end of calling any f’n address, it is called binding process.
- With the help of binding process, compiler will recognize that which f’n need to be called at the time of execution.
void main() // address of xyz
{ printf("\n Hello to xyz”); }
void abc() // & abc
{ printf(“\nHello abc”); xyz(); // calling &xyz }
void main() //& main
{ printf(“\n Hello main”); xyz(); //calling &xyz() abc(); // calling &abc(); } o/p : Hello main Hello xyz Hello abc Hello xyz
- When we are working with the f’ns, it is possible to implement randomly, i.e in any sequence , f’ns can be implemented.
- From any f’ns, we can invoke any other f’n
- After execution of any f’n, automatically control will pass back to the calling location, i.e , from which place we called the f’n , to come place it will pass
void main()
{ printf(“\n welcome main”); } void abc() { printf(“\n welcome abc”); } o/p : Type mismatch in redeclaration of ‘abc’
void abc()
{ } void main() Parameter - > void { No of parameters abc(); } 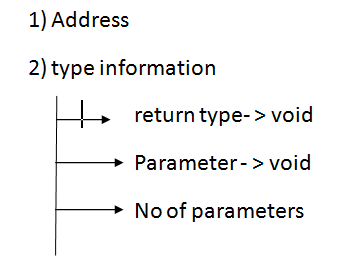
- Whenever the f’n is compiler before calling then along with the address, type information will maintain by compiler, i.e return type, parameter type and no.of parameters.
- Whenever the f’n is comiling after calling then automatically compiler will main default type information i.e return type as an int, parameter type is void and no.of parameters are zero.
- In above program, compiler is expecting the return type as an int, but actually return type is void. So , mismatch will occur
- In implementation , whenever we are calling a f’n which is defined later, then for avoiding the compilation error, we required to go for prototype, i.e forward declaration is required.
- Declaration of f’n is required to specify return type. name of the fu’n and parameter type information.
- Forward declaration statement will provides type information explicitly so, compiler doesn’t takes any default information
void main()
{ void abc(void); //declaration // void abc(); printf(“\n welcome main”); abc(); }
void abc()
{ printf(“\n welcome abc”); } o/p : welcome main welcome abc
void abc()
{ printf(“\n welcome abc”); xyz(); }
void main()
{ printf(“\n Hello main”); xyz(); abc(); }
void xyz()
{ printf(“\n welcome xyz”); } o/p : Error type mismatch in redeclaration of ‘xyz’
- In order to call the xyz f’n in abc and main we require to go for forward declaration of xyz in abc and main also.
- In implementation when we require to provide the forward declaration of a f’n more than once then recommended to go for global declaration
- Whenever we are declaring a f’n top of a program before defining the first f’n then it is called global declaration
- If the global declaration is available. then doesn’t required to go for local declaration. IF local declaration also available, then global declaration will ignore.
void xyz(); // global declaration
void abc(); { // void xyz(); // local declaration printf(“\n welcome abc”); xyz(); } void main() { // void xyz(); local declaration printf(“/n hello main”); xyz(); abc(); } void xyz() { printf(“\n welcome xyz”); } o/p : Hello main Welcome xyz Welcome abc; Welcome xyz;